Using Redux with Axios in Your React App
Redux is a predictable state management library commonly used with React applications. Axios is a popular JavaScript library used to make HTTP requests from the browser (or Node.js).
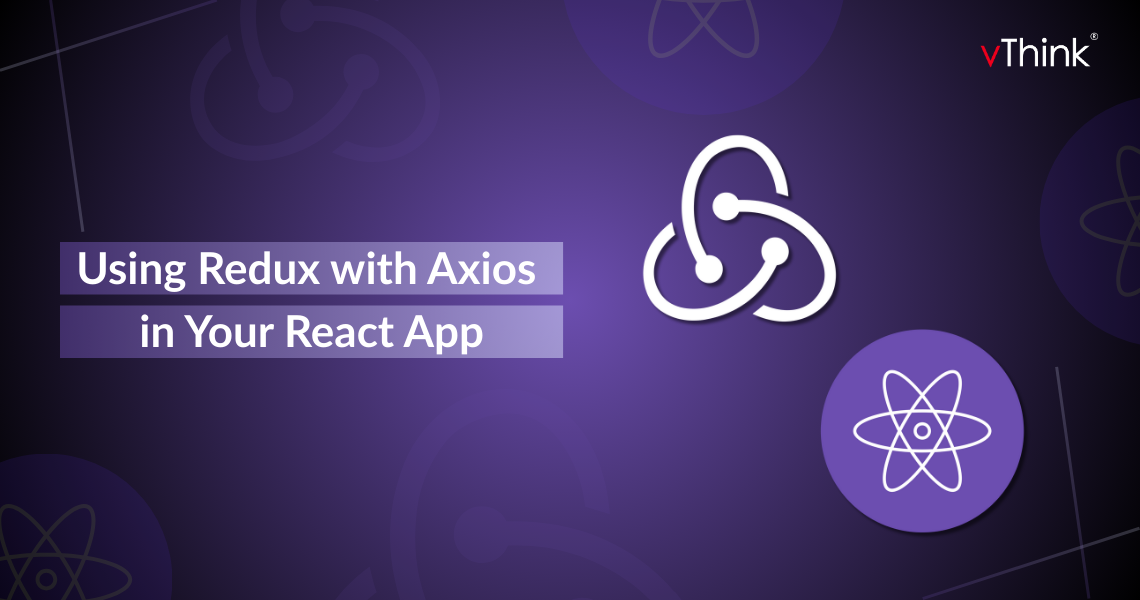
Redux
What is Redux?
Redux is a predictable state management library commonly used with React applications. It helps manage the global state of an application in a centralized store, allowing components to access and update state in a consistent and controlled manner.
Why Redux is Used in React Applications?
In small React apps, managing state through local component state and prop drilling (passing props from parent to child) is usually sufficient. However, in larger applications, passing data through many layers of nested components becomes cumbersome and error-prone. This is where Redux becomes valuable.
How Redux Helps?
- Centralized Store: Redux maintains a single source of truth for the entire application's state, making it easier to track and debug.
- Predictable State Updates: State changes in Redux are handled by pure functions called reducers, which ensure that updates are consistent and traceable.
- Improved Scalability: By avoiding deep prop drilling, Redux allows any component to access the state it needs directly from the store, improving scalability and maintainability.
Redux Core Concepts
Store
- The store is the central hub of Redux. It holds the entire application state as a single immutable object. The store provides methods to:
- Retrieve the current state (getState()).
- Dispatch actions to update the state (dispatch(action)).
- Subscribe to state changes (subscribe(listener))
Actions
- An action is a plain JavaScript object that describes an event in the application. Every action must have a type property that defines the event type. It may also include additional data (payload).
- Actions are the only way to modify the state in Redux. They are usually created using action creators, which are functions that return action objects.
- Example actions could be:
const addItem = (item) => {
return {
type: 'ADD_ITEM',
payload: item
};
};
Reducers
- A reducer is a pure function that determines how the state should change in response to an action.
- Take the current state and an action as arguments.
- Return a new state based on the action type.
const initialState = { items: [] };
const listReducer = (state = initialState, action) => {
switch (action.type) {
case 'ADD_ITEM':
return { ...state, items: [...state.items, action.payload] };
case 'REMOVE_ITEM':
return { ...state, items: state.items.filter(item => item !== action.payload) };
default:
return state;
}
};
// Example state updates
console.log(listReducer(undefined, { type: 'ADD_ITEM', payload: 'Learn Redux' }));
// Output: { items: ['Learn Redux'] }
Dispatch
- The dispatch(action) method is used to send an action to the store. The store then passes the action to the reducer, which updates the state accordingly.
- Dispatching is the only way to change the state in Redux. Components use dispatch() to trigger state updates.
Subscribe
- The subscribe(listener) method allows external code to listen for state updates. Whenever the state changes, the subscribed function executes.
- This is useful for:
UI updates: Re-rendering components when the state changes.
Logging state changes for debugging.
Persisting state changes (e.g., saving state to local storage).
const addItem = (item) => ({
type: 'ADD_ITEM',
payload: item
});
const itemsReducer = (state = [], action) => {
switch (action.type) {
case 'ADD_ITEM':
return [...state, action.payload];
default:
return state;
}
};
Setting Up Redux in a React Project
Install Redux and React-Redux
- Command for installing:
npm install redux react-redux
Create Redux Store
- Show how to create and configure the store using createStore().
- Example: Setting up a simple store with a reducer.
Connecting Redux to React
- Use Provider from react-redux to connect the store to the React app.
- Example: Wrapping the root component with Provider.
React-Redux Hooks (useSelector and useDispatch)
useSelector
- How to get the current state from the Redux store.
- Example of selecting the list of items from the Redux state.
useDispatch
- How to dispatch actions from React components.
- Example of dispatching an action to add an item.
Code Example
import { useDispatch, useSelector } from 'react-redux';
const ItemsList = () => {
const items = useSelector(state => state.items);
const dispatch = useDispatch();
const addItem = (item) => {
dispatch({ type: 'ADD_ITEM', payload: item });
};
return (
<div>
<button onClick={() => addItem('New Item')}>Add Item</button>
<ul>
{items.map((item, index) => (
<li key={index}>{item}</li>
))}
</ul>
</div>
);
};
Axios
What is Axios?
Axios is a popular JavaScript library used to make HTTP requests from the browser (or Node.js). In React applications, Axios is commonly used to communicate with backend APIs, such as fetching data or sending user input to a server.
Why is Axios Used in React Applications?
React itself does not include built-in functionality for making HTTP requests. While native options like fetch exist, Axios provides a simpler and more powerful API with added features that make it a preferred choice.
How Axios Helps?
- Simplified Syntax: Axios uses a cleaner, promise-based syntax that’s easier to work with compared to fetch, especially when dealing with JSON.
- Automatic JSON Parsing: Responses are automatically converted to JSON, reducing boilerplate code.
- Request and Response Interceptors: Allows developers to modify requests or handle errors in a centralized way (e.g., adding auth tokens).
- Error Handling: Provides more consistent and informative error responses than fetch.
- Support for Older Browsers: Axios includes features that work more reliably across different browsers.
Installing Axios
Install Axios using npm/yarn:
npm install axios
(or)
yarn add axios
Making Basic HTTP Requests with Axios
GET Request
- How to fetch data using the axios.get() method.
- Example: Fetching data from an API.
import axios from 'axios';
axios.get('https://jsonplaceholder.typicode.com/posts')
.then(response => {
console.log('Data:', response.data);
})
.catch(error => {
console.log('Error:', error);
});
POST Request
- How to send data using the axios.post() method.
- Example: Sending form data to the server.
const postData = { title: 'New Post', body: 'This is a new post' };
axios.post('https://jsonplaceholder.typicode.com/posts', postData)
.then(response => {
console.log('Post Created:', response.data);
})
.catch(error => {
console.log('Error:', error);
})
PUT and DELETE Requests
- Examples of PUT (updating data) and DELETE (removing data).
// PUT Request
const updatedData = { title: 'Updated Post', body: 'This post has been updated' };
axios.put('https://jsonplaceholder.typicode.com/posts/1', updatedData)
.then(response => {
console.log('Post Updated:', response.data);
});
// DELETE Request
axios.delete('https://jsonplaceholder.typicode.com/posts/1')
.then(response => {
console.log('Post Deleted:', response.status);
})
Handling Responses and Errors
Handling Response Data
- Use .then() and .catch() to handle success and failure.
- The response object contains properties like data, status, and headers.
Handling Errors
- How to handle errors using .catch().
- You can check for different types of errors (e.g., network errors, 404s, 500s, etc.).
axios.get('https://jsonplaceholder.typicode.com/invalid-url')
.then(response => {
console.log('Data:', response.data);
})
.catch(error => {
if (error.response) {
// Server responded with a status other than 2xx
console.error('Error Response:', error.response.data);
} else if (error.request) {
// Request was made but no response was received
console.error('Error Request:', error.request);
} else {
// Something else caused the error
console.error('Error Message:', error.message);
}
});
Using Axios with async/await
Simplified syntax
- Using async/await to make code cleaner and easier to understand.
const fetchData = async () => {
try {
const response = await axios.get('https://jsonplaceholder.typicode.com/posts');
console.log('Data:', response.data);
} catch (error) {
console.error('Error:', error);
}
};
fetchData();
Customizing Axios Requests
Setting Headers
- How to customize headers (e.g., for authentication tokens).
const config = {
headers: {
'Authorization': 'Bearer your_token_here',
}
};
axios.get('https://jsonplaceholder.typicode.com/posts', config)
.then(response => {
console.log('Data with Authorization:', response.data);
});
Setting Base URL
- You can create an Axios instance with a base URL so you don’t need to repeat it in every request.
const api = axios.create({
baseURL: 'https://jsonplaceholder.typicode.com'
});
api.get('/posts')
.then(response => {
console.log('Data from API:', response.data);
});
Interceptors
What are Axios interceptors?
- Interceptors allow you to run code or modify the request or response before it is handled by .then() or .catch().
Request Interceptor
- Example of adding a token to the header before sending a request.
axios.interceptors.request.use(
config => {
// Add authorization token to headers
config.headers['Authorization'] = 'Bearer your_token_here';
return config;
},
error => {
return Promise.reject(error);
}
);
Response Interceptor
- Example of handling global error responses.
axios.interceptors.response.use(
response => {
return response;
},
error => {
if (error.response.status === 404) {
console.error('Page not found');
}
return Promise.reject(error);
}
);
Cancelling Requests
Why and when to cancel requests?
- Cancelling requests is useful in scenarios like when a user navigates away before the request finishes.
- Example using CancelToken:
axios.get(' cancelToken: cancelTokenSource.token
})
.then(response => {
console.log('Data:', response.data);
})
.catch(error => {
if (axios.isCancel(error)) {
console.log('Request cancelled', error.message);
} else {
console.log('Error:', error);
}
});
Handling Timeouts
- Set a timeout to avoid hanging requests.
axios.get('https://jsonplaceholder.typicode.com/posts', { timeout: 5000 })
.then(response => console.log(response.data))
.catch(error => console.error('Timeout Error:', error));
Parallel Requests
- Make multiple requests at once using axios.all().
axios.all([
axios.get('https://jsonplaceholder.typicode.com/users'),
axios.get('https://jsonplaceholder.typicode.com/posts')
])
.then(axios.spread((users, posts) => {
console.log('Users:', users.data);
console.log('Posts:', posts.data);
}))
.catch(error => console.error('Error:', error));